What is Cross Site Scripting, how does it work, and how can developers prevent it? Learn here.
Preface
If you ever used a website or an application, chances are that you’ve come across a search form or two. Search forms aren’t anything unusual – most of them record searches in a database, then display them underneath the form, in a comments section, or elsewhere.
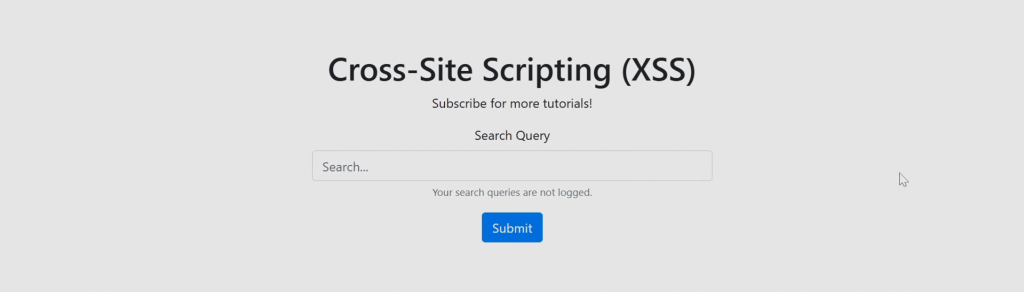
Search forms like the one above usually work like so:
- User input is provided via an entry into the form.
- User input is usually saved in a database.
- The application takes the information provided by the user or saved in a database (step #2) and displays that information on the screen.
- User input can be acted on further if necessary (i.e. modified or deleted according to some rules, etc.)
That’s nothing unusual – what’s dangerous within these steps though is the fact that frequently, use input is displayed to the user or other users without any form of validation, making the application susceptible to cross site scripting, or XSS.
What is Cross Site Scripting?
Cross site Scripting is a type of attack directed towards applications. Cross site Scripting, or XSS for short, can take multiple forms depending on how the data in question is handled. We can have:
- Reflected Cross-site Scripting where user input is “reflected” (displayed) back to the user (most likely via an HTTP request.)
- Stored Cross-site Scripting where user input is stored in a database and then displayed to the user or other users from the database.
- DOM-based Cross-site Scripting where the vulnerability exists in client-side code and affects the Document Object Model (DOM.)
Usually, the type of Cross-Site Scripting an application is vulnerable to is quite easy to identify: reflected cross site scripting will “reflect” – display – the user input back to the user once it is provided and won’t store it anywhere (i.e. upon another visit to the same webpage the input provided won’t be visible anymore), stored cross site scripting will store the input provided by a user in a database and that can also be very easily identified because in that case, user input (or even a list of user inputs like in the example below) will be displayed under the search form, in a specialized box, or elsewhere:
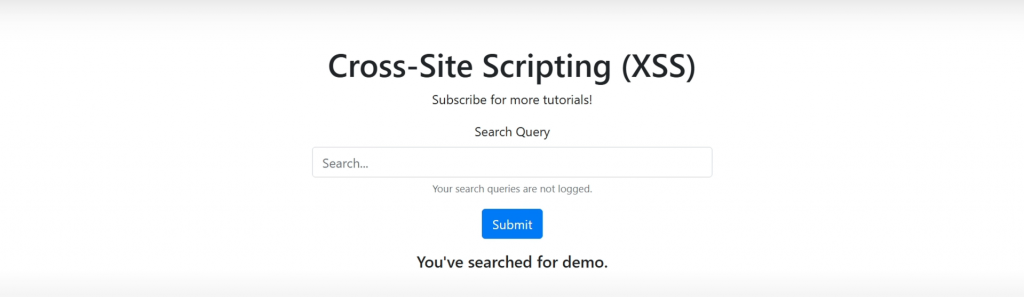
The problem with Cross Site Scripting is that anyone with enough knowledge of JavaScript can craft a malicious payload and steal session cookies, craft redirects, or trick users into providing sensitive information. That’s the primary reason Cross Site Scripting was one of the most popular attack vectors for OWASP ever since 2010 – the 2021 edition of OWASP Top 10 seemingly included the attack inside the Injection category, and it’s very likely to be included in the upcoming edition of OWASP as well. The type of XSS in use has a very big part of the game to play here – stored XSS is likely to be more dangerous than reflected simply because reflected Cross Site Scripting isn’t saved anywhere but don’t be fooled: it can still be passed to a user via a link or by other means.
Finding & Remediating Cross Site Scripting
Thankfully, finding Cross Site Scripting vulnerabilities in your code is rather simple. Look for:
- Unfiltered user input being written into a database and then displayed via an application
- User input being provided and displayed in a part of an application
Also, keep in mind that applications can lie to you. As you can see, this part of the application was a lie – search queries were being logged…:

When probable XSS is found (you will see for yourself – finding it is quite easy given you have an adequate amount of experience), wrap it around htmlentities, htmlspecialchars, or equivalent functions, and you should be good to go because you will no longer display user input to the user!
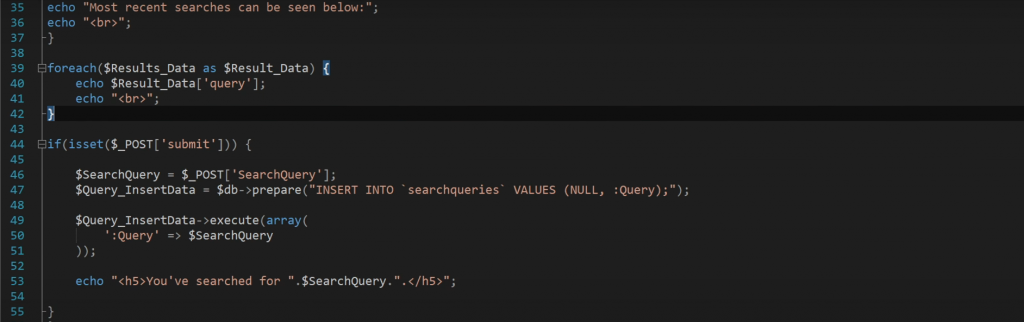
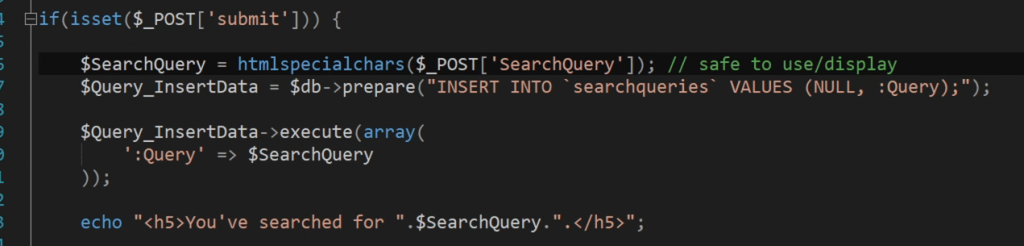
The point of you doing this is to turn every HTML character (those will run the Javascript necessary for XSS to execute) into a „harmless“ HTML equivalent: e.g. „<“ will become „<“ (less than), „>“ will become „>“ (greater than), etc.
As such, Cross site Scripting will no longer be one of your worries:
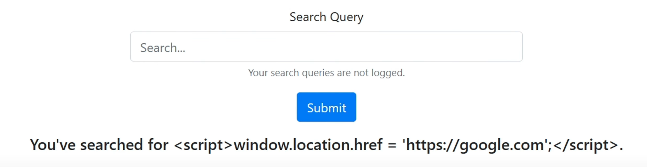
Of course, Cross Site Scripting is only one attack you need to care about. It‘s just a tip of the iceberg as there are more – keeping yourself up to date with OWASP Top 10 should set you on the right path. For now, dig into a YouTube video depicting Cross Site Scripting if you‘d prefer a visual explanation:
Protecting Yourself with Data Breach Search Engines
Once application-based attacks are out of the way, it‘s time to think about the way you protect your most precious data aside from them. Using data breach search engines is a great way to do that:
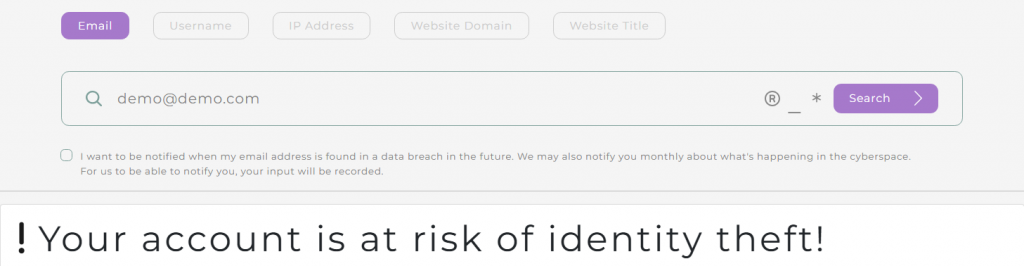
Data breach search engines like BreachDirectory will let you search for your email address, username, IP address, or other details, and if these details occur in any data breaches that are archived in its database, it will provide you with a warning. Most data breach search engines also provide you with an API capability letting you obtain the information from their database and use it for your use case: BreachDirectory API is also one of them. Take the BreachDirectory API a spin today, stay safe from Cross Site Scripting and other types of attacks by sharing this blog post (and a link to the data breach search engine) with your friends, and until next time!
FAQ
What is Cross Site Scripting?
Cross site scripting, or XSS for short, is a type of attack directed at applications that aims to execute Javascript code via an application to harm a user by impersonating him, stealing his cookies, crafting malicious redirects, or performing other actions. Learn more about Cross site Scripting and its types here.
What are the types of Cross Site Scripting?
Cross site scripting has three types: it can be reflected (displayed to the user), stored (stored in a database), or DOM-based.
How Can I Protect My Application from Cross Site Scripting?
You can protect your application from XSS by escaping HTML characters by using functions like htmlspecialchars, htmlentities, or other functions.